This feature is available only with paid Kochava accounts. Contact us to learn more.
If you have already integrated the SDK and started it, please visit Using the SDK support page and choose a topic.
Integrating the SDK
Requirements:
- NodeJS
- NPM
- Angular
Supported Platforms:
- Angular
Data Privacy:
Integration:
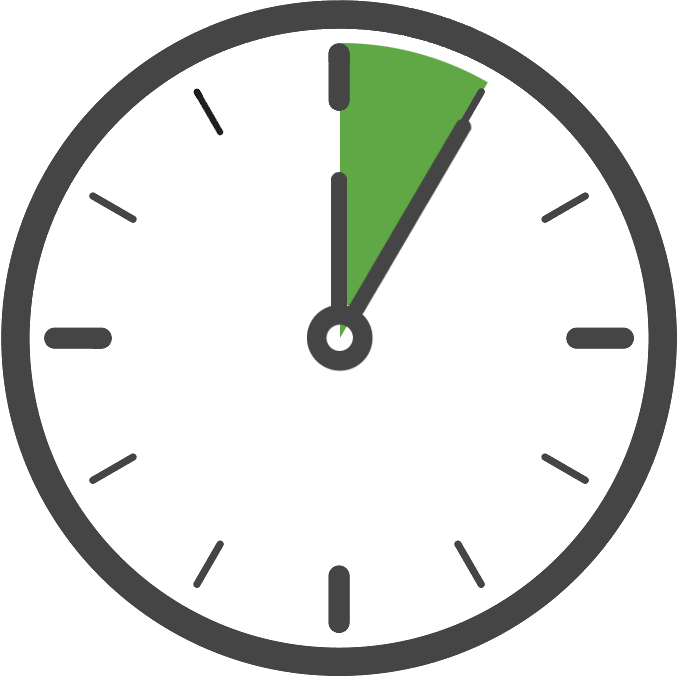
The Kochava Web SDK is available as a first-class Angular library. The SDK itself operates as an Angular service singleton, available within the KochavaAngularModule.
- Navigate to the root of your Angular project.
- Run
ng add kochava-angular
. - Run
npm install kochava@latest
. - In your root component’s module file (typically app.module.ts) add the following:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { KochavaAngularModule } from 'kochava-angular'; // import the module
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
KochavaAngularModule.forRoot() // call forRoot() on the module
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Starting the SDK
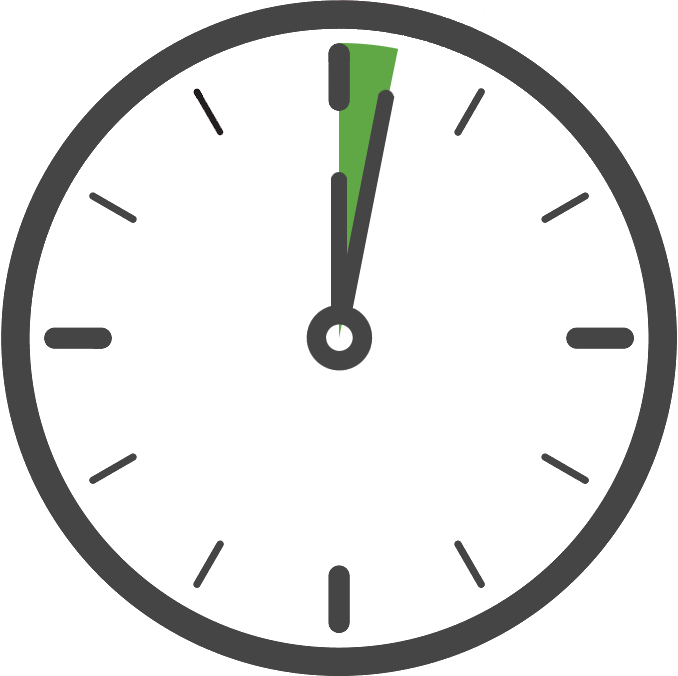
Once you have added the Kochava SDK to your project, the next step is to create and start the SDK class in code. Only your App GUID is required to start the SDK with the default settings, which is the case for typical integrations.
Kochava recommends starting the SDK as soon as the application starts, although this can be done later if needed. Starting the tracker as early as possible will ensure it has started before use, resulting in more accurate data/behavior.
- In your root component (usually app.component.ts), import the KochavaAngularService and inject it into the constructor. Then call startWithAppGuid with your Kochava app guid.
- Replace
YOUR_APP_GUID
with your Kochava App GUID. For more information on locating your App GUID, refer to our Locating your App GUID support documentation. - For any additional components which use the SDK, import the KochavaAngularService and inject it as a dependency into the component’s constructor. No additional provider entries are required.
import { Component } from '@angular/core';
import { KochavaAngularService } from 'kochava-angular'; // import the service
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
providers: [
KochavaAngularService // add the service as a provider
]
})
export class AppComponent {
title = 'angular';
// inject service in the constructor
// you can call the service whatever you would like instead of 'kos'
constructor(kos: KochavaAngularService) {
// start the SDK
// replace YOUR_APP_GUID with your kochava app guid
kos.kochava.startWithAppGuid("YOUR_APP_GUID");
}
}
import { KochavaAngularService } from 'kochava-angular';
...
export class AppComponent {
constructor(kos: KochavaAngularService) {
kos.kochava.sendPageEvent("test_page"); // example of page
}
}
NOTE: These calls are optional and used for irregular SDK behavior. In most cases, you do not need to call these functions at all.
- Disable automatic page events – Call this function with an argument of true to stop the SDK from automatically signaling a page event when the SDK starts.
- Use cookie storage – Call this function with an argument of true to drop the Cookie on the website to track a device across sub-domains.
import { Component } from '@angular/core';
import { KochavaAngularService } from 'kochava-angular';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
providers: [
KochavaAngularService
]
})
export class AppComponent {
title = 'angular';
kos: KochavaAngularService;
constructor(kos: KochavaAngularService) {
this.kos = kos;
// automatic page events will be sent out (default)
this.kos.kochava.disableAutoPage(false);
// automatic page events will not be sent out
this.kos.kochava.disableAutoPage(true);
// cookies will not be used for sdk storage (default)
this.kos.kochava.useCookies(false);
// cookies will be used for sdk storage
this.kos.kochava.useCookies(true);
this.kos.kochava.startWithAppGuid("kowebsdkv3-7pv0");
}
}
Confirm the Integration
Where to Go From Here:
Now that you have completed integration you are ready to utilize the many features offered by the Kochava SDK. Continue on to Using the SDK and choose a topic.